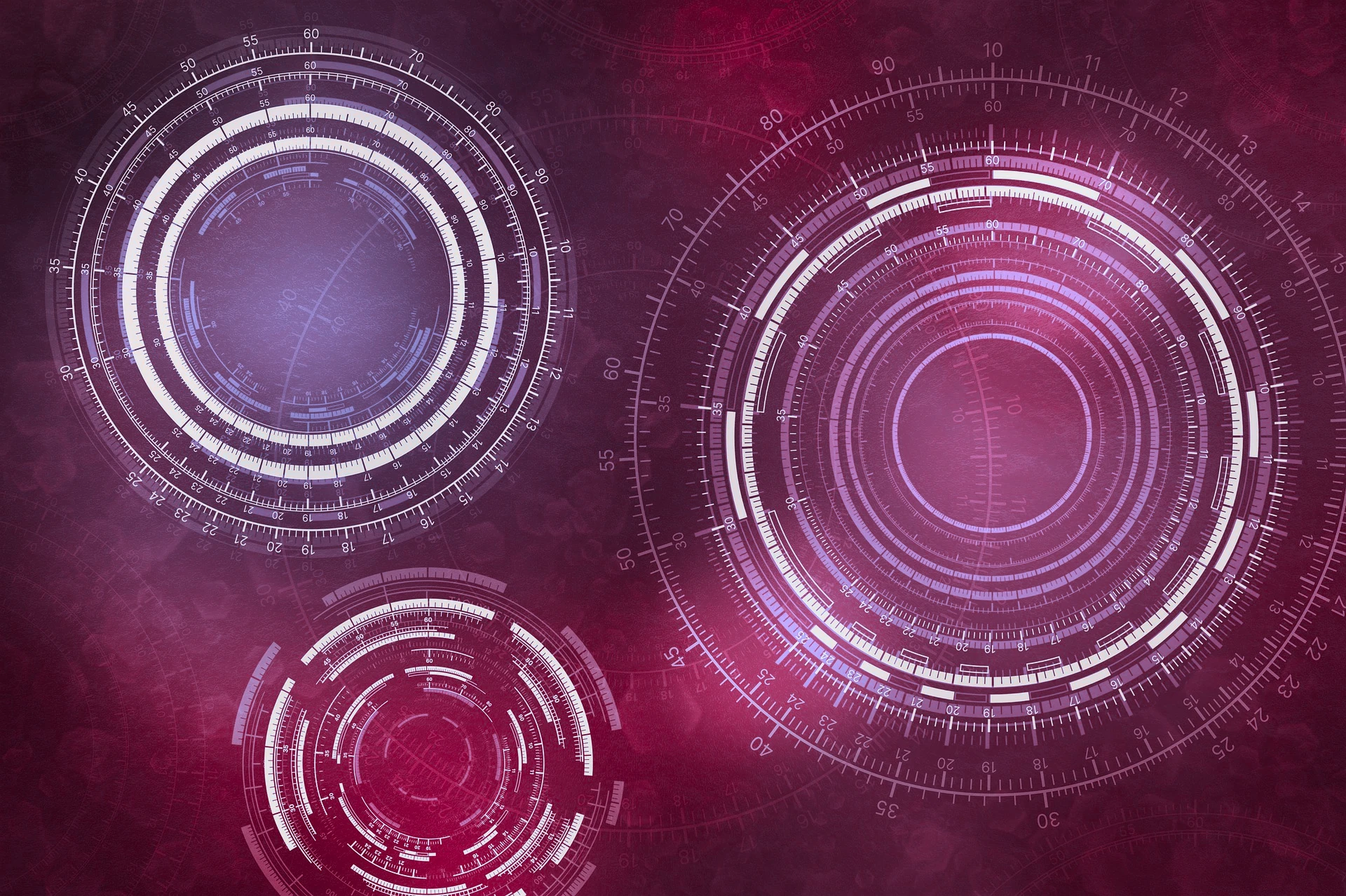
Node.js Essentials
Initialize project with metadata (package.json
)
npm init -y
Adding dev dependencies. -D signifies that this package is a development dependency and will show up under the devDependecies section in package.json. This means that the package will only be included in development mode and will not be included in the production bundle.
npm install <package> -D
Import/Export in JavaScript: Node.js vs ES6
There is a difference in how you import or export based on whether you are in the Node runtime or in the browser.
- The Node runtime environment and the
module.exports
andrequire()
syntax.
module.exports = functionName;
- The browser’s runtime environment and the ES6
import
/export
syntax.
Error Handling with Async Code
When Error Handling asynchronous code, it is not good to use try…catch blocks as the error may never be caught. In the case of async code, then, it is better to use “if (err)…else” block. An error first callback.
let errorFirstCallback = (err, data) => {
if (err) {
console.log(`Something went wrong. ${err}\n`);
} else {
console.log(`Something went right. Data: ${data}\n`);
}
};
api.errorProneAsyncApi("problematic input", errorFirstCallback);
Events (eventEmitter
module)
In order to attach a callback function to a named event, use the .on()
method.
Buffer Module (Buffer
module)
A global object that is used to represent a dynamic amount of memory that can’t be resized. Handles binary data. Turning JavaScript objects and entities into bytes and vice versa. Can also concatenate binary data together and then output the result. No need to import.
const bufferAlloc = Buffer.alloc(15, 'b') // creates fifteen b's
const buffer1 = Buffer.from("hello");
const buffer2 = Buffer.from("world");
const bufferConcat = [buffer1, buffer2];
Buffer.concat(bufferConcat);
File System (fs
module)
Reads and writes files on local machine.
const fs = require('fs');
let secretWord = "cheeseburgerpizzabagels";
let readDataCallback = (err, data) => {
if (err) {
console.log(`Something went wrong: ${err}`);
} else {
console.log(`Provided file contained: ${data}`);
}
};
fs.readFile("./finalFile.txt", "utf-8", readDataCallback);
Streaming Data (readline
module)
Reading or writing data piece by piece.
Example of reading data via stream:
const readline = require('readline');
const fs = require('fs');
const myInterface = readline.createInterface({
input: fs.createReadStream('text.txt')
});
myInterface.on('line', (fileLine) => {
console.log(`The line read: ${fileLine}`);
});
// alternatively
function printData (data) {
console.log(`Item: ${data}`)
}
myInterface.on("line", printData);
Example of writing data using emitter based on reading the contents of another file:
const myInterface = readline.createInterface({
input: fs.createReadStream('shoppingList.txt')
});
const fileStream = fs.createWriteStream("shoppingResults.txt");
function transformData(line) {
fileStream.write(`They were out of: ${line}\n`)
}
myInterface.on("line", transformData);
Time (timer
module)
Includes setTimeout()
and setInterval()
.
Using setImmediate()
to execute a function after the current (poll phase) is completed:
setImmediate(() => {
console.log("I got called right away!")
})
© Filip Niklas 2024. All poetry rights reserved. Permission is hereby granted to freely copy and use notes about programming and any code.