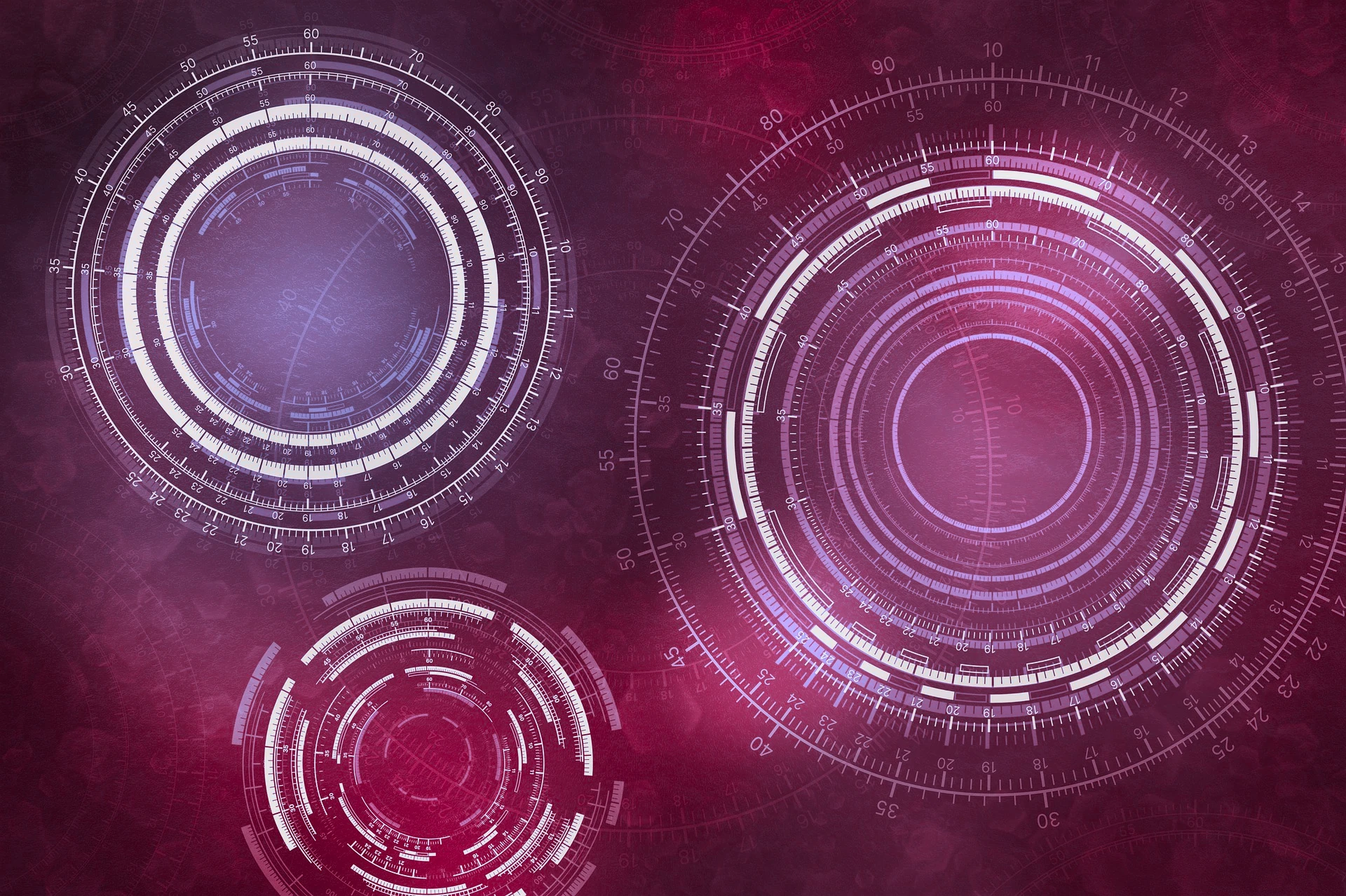
Simple Express.js server that responds to basic HTTP methods.
const express = require('express');
const app = express();
// helper functions
const { getElementById, getIndexById, updateElement, seedElements, createElement } = require('./utils');
const animals = [];
seedElements(animals, 'animals');
const PORT = process.env.PORT || 4001;
// GET (read)
app.get('/animals', (req, res, next) => {
res.send(animals);
});
// GET specific
app.get('/animals/:id', (req, res, next) => {
const foundExpression = getElementById(req.params.id, animals);
if (foundExpression) {
res.send(foundExpression);
} else {
res.status(404).send();
}
});
// PUT (update)
app.put('/animals/:id', (req, res, next) => {
const expressionIndex = getIndexById(req.params.id, animals);
if (expressionIndex !== -1) {
updateElement(req.params.id, req.query, animals);
res.send(animals[expressionIndex]);
} else {
res.status(404).send();
}
});
// POST (create)
app.post('/animals', (req, res, next) => {
const receivedExpression = createElement('animals', req.query);
if (receivedExpression) {
animals.push(receivedExpression);
res.status(201).send(receivedExpression);
} else {
res.status(400).send();
}
});
// DELETE
app.delete('/animals/:id', (req, res, next) => {
const expressionIndex = getIndexById(req.params.id, animals);
if (expressionIndex !== -1) {
animals.splice(expressionIndex, 1);
res.status(204).send();
} else {
res.status(404).send();
}
});
// server listen
app.listen(PORT, () => {
console.log(`Listening on port ${PORT}`);
});
Grouping routes into routers and then refactoring code to address this.
const expressionsRouter = express.Router();
app.use("/expressions", expressionsRouter);
// Get all expressions
// NOTICE that the path here is simply "/" since its specific endpoint is already defined at the router level.
expressionsRouter.get('/', (req, res, next) => {
res.send(expressions);
});
© Filip Niklas 2024. All poetry rights reserved. Permission is hereby granted to freely copy and use notes about programming and any code.